- Total
꿈꾸는리버리
FileManager로 파일 읽고 쓰기 + 폴더 만들기 본문
앞서 블로그에서 확인한 것처럼
numbers에 있는 내용을 txt 파일로 변환을 해서 폴더로 만들어야 했다..
해야 하는 일들을 list화 시키면 다음과 같다.
- numbers의 내용을 json으로 변환하고
- txt 파일 내의 json의 내용을 읽어내고
- json의 내용을 string -> struct으로 변환
- struct으로 변환한 json의 Text 내용은 txt 파일에 넣고 이 txt 파일을 Emotion folder으로 넣어 분류해야 한다.
1. numbers의 내용을 json으로 변환하고
이 부분은 이미 전에 해봤던 거기 때문에 간단하게 할 수 있었다 ! ( 해당 블로그 포스팅 참고 )
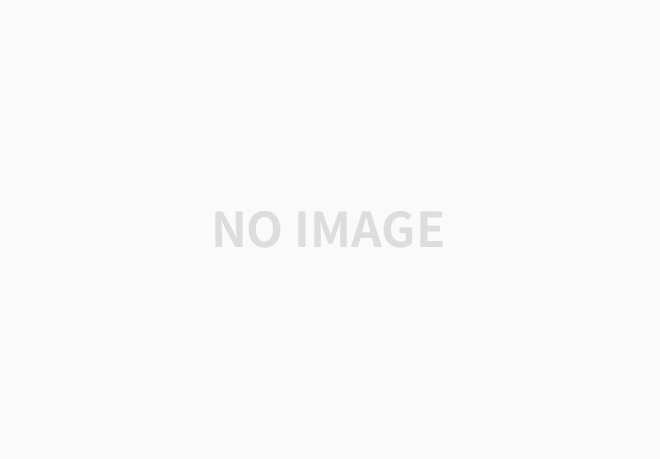
2. txt 파일 읽기
이때부터가 어려움의 시작이었다. 처음에는 어차피 파일만 만들고 필요없어지는 코드라고 생각했기 때문에, json을 복사해서 변수로 넣으려고 했는데.. ㅋㅋㅋ 복붙을 할 때마다 양이 많아서 그런가.. 무한 로딩이 걸렸다.
그래서.. txt 파일에 있는 json을 읽어오는 코드가 필요했다.
var emotionString = ""
do {
// URL을 불러와서 Data타입으로
let dataFromPath: Data = try Data(contentsOf: URL(string: "json.txt 파일 경로")!)
// Data -> String
emotionString = String(data: dataFromPath, encoding: .utf8) ?? "문서없음"
} catch let e {
print(e.localizedDescription)
}
요로케 하면 emotionString에 해당 txt 파일 안에 있는 json이 읽어졌다.
3. json의 내용을 string -> struct으로 변환
struct Emotion: Codable {
let Text: String?
let Emotion: String?
}
if let json = emotionString.data(using: .utf8) {
let decodedItems = try JSONDecoder().decode([Emotion].self, from: json)
}
4. struct으로 변환한 json의 Text 내용은 txt 파일에 넣고 이 txt 파일을 Emotion folder으로 넣어 분류
문서 폴더 안에는 필요한 폴더들을 넣어놨다 ( 아래 함수에서 folderName인 애들 )
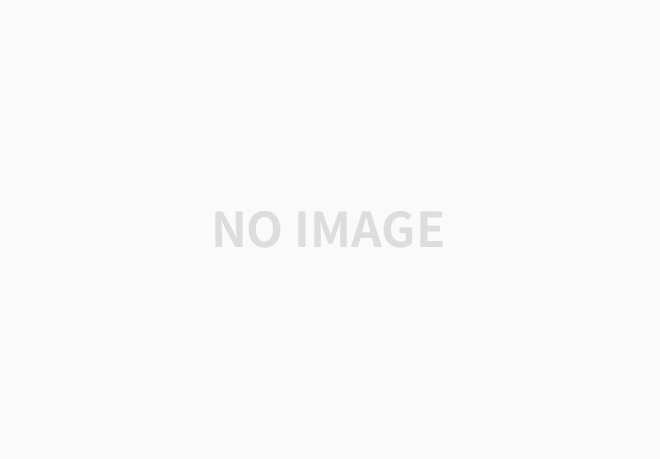
만약 문서 경로가 어딘지 모르겠다면, 우측의 URL을 복사해서 검색하면 finder에서 찾아낼 수 있다.
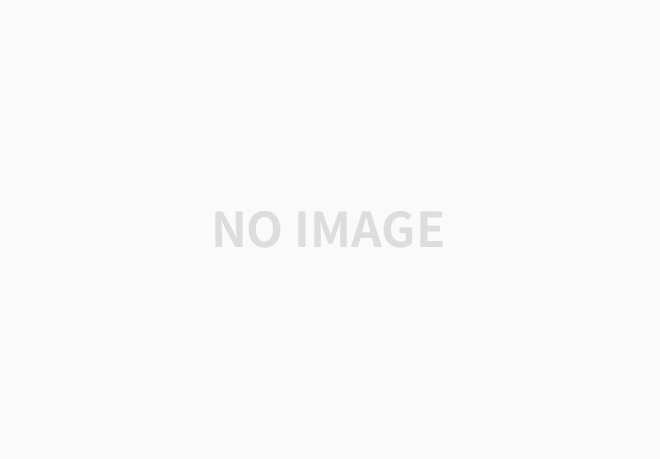
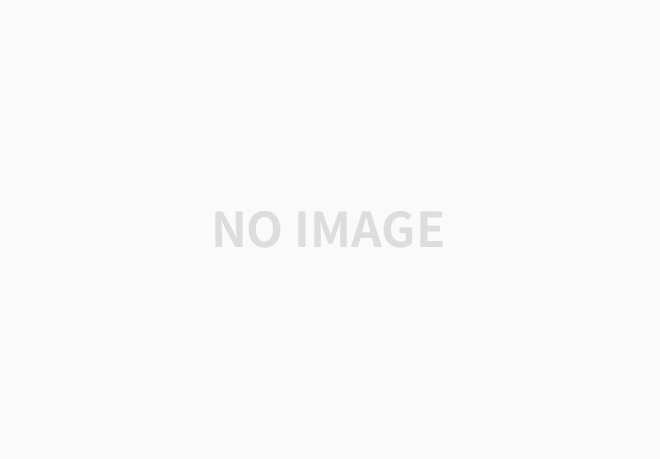
func makeFile(number: Int, folderName: String, content: String) {
// FileManager 인스턴스 생성하기
let fileManager: FileManager = FileManager.default
// 사용자의 문서 경로
let documentPath: URL = fileManager.urls(for: .documentDirectory, in: .userDomainMask)[0]
// 사용자의 문서 경로에 있는 디렉토리를 찾아 디렉토리 경로(URL) 반환
let directoryPath: URL = documentPath.appendingPathComponent(folderName)
// folderName 디렉토리 안에 만들 'xx.txt'의 경로 찾기
let textPath: URL = directoryPath.appendingPathComponent("\(folderName)_\(number).txt")
// 아까 만든 'xx.txt' 경로에 텍스트 쓰기
// content String을 Data로 변환
if let data: Data = content.data(using: String.Encoding.utf8) {
do {
// 변환한 data를 "xx.txt"에 쓰기
try data.write(to: textPath)
} catch let e {
print(e.localizedDescription)
}
}
}
그리고 emotionString을 struct으로 변환했던 부분에 해당 함수를 반복문으로 호출시켜줬다.
if let json = emotionString.data(using: .utf8) {
let decodedItems = try JSONDecoder().decode([Emotion].self, from: json)
for item in decodedItems {
makeFile(number: number, folderName: item.Emotion!, content: item.Text!)
number += 1
}
}
그러니 생겨난.. 이 귀여운 녀석들 😭

전체 코드
import Foundation
func makeFile(number: Int, folderName: String, content: String) {
// FileManager 인스턴스 생성하기
let fileManager: FileManager = FileManager.default
// 사용자의 문서 경로
let documentPath: URL = fileManager.urls(for: .documentDirectory, in: .userDomainMask)[0]
// 사용자의 문서 경로에 있는 디렉토리를 찾아 디렉토리 경로(URL) 반환
let directoryPath: URL = documentPath.appendingPathComponent(folderName)
// 파일매니저로 디렉토리 생성하기
// do {
// // 아까 만든 디렉토리 경로에 디렉토리 생성
// try fileManager.createDirectory(at: directoryPath, withIntermediateDirectories: false, attributes: nil)
// } catch let e {
// print(e.localizedDescription)
// }
// folderName 디렉토리 안에 만들 'xx.txt'의 경로 찾기
let textPath: URL = directoryPath.appendingPathComponent("\(folderName)_\(number).txt")
// 아까 만든 'xx.txt' 경로에 텍스트 쓰기
// content String을 Data로 변환
if let data: Data = content.data(using: String.Encoding.utf8) {
do {
// 변환한 data를 "xx.txt"에 쓰기
try data.write(to: textPath)
} catch let e {
print(e.localizedDescription)
}
}
}
// 만든 파일 불러와서 읽기.
var emotionString = ""
do {
// URL을 불러와서 Data타입으로
let dataFromPath: Data = try Data(contentsOf: URL(string: "json.txt 파일 경로")!)
// Data -> String
emotionString = String(data: dataFromPath, encoding: .utf8) ?? "문서없음"
} catch let e {
print(e.localizedDescription)
}
var number = 0
struct Emotion: Codable {
let Text: String?
let Emotion: String?
}
if let json = emotionString.data(using: .utf8) {
let decodedItems = try JSONDecoder().decode([Emotion].self, from: json)
for item in decodedItems {
makeFile(number: number, folderName: item.Emotion!, content: item.Text!)
number += 1
}
}
참고한 문서
https://zeddios.tistory.com/440
iOS ) FileManager를 이용해 파일/폴더 만드는 법
안녕하세요 :) Zedd입니다. 제목이 뭔가 추상적인데.....이 글은...저를 위한....공부... FileManager를 이용해 파일/폴더 만드는 법 파일 및 디렉토리와 관련된 가장 기본적인 작업 중 일부는 파일 시스
zeddios.tistory.com
https://developer.apple.com/documentation/foundation/jsondecoder
Apple Developer Documentation
developer.apple.com
The JSON Validator
JSONLint is the free online validator and reformatter tool for JSON, a lightweight data-interchange format.
jsonlint.com
'오뚝이 개발자 > swift' 카테고리의 다른 글
모나드란? with Context / Functor (0) | 2024.03.21 |
---|---|
Swift) Dictionary 초기화 방법 (2) | 2023.05.12 |
DateFormatter extension으로 관리하기 (0) | 2022.07.25 |
delegate란? (protocol을 곁들인..) (1/2) (0) | 2022.07.24 |
protocol 정복기 ( 3 / 3) (0) | 2022.07.24 |